mirror of
https://github.com/netzbegruenung/jitsi-meet-electron.git
synced 2024-04-30 07:44:53 +02:00
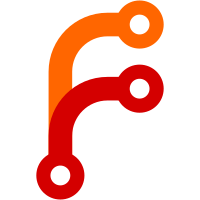
In some OS/Chromium combinations the automatic gain control goes slightly crazy, but normally its fine. Thus keep the default as is, but add an option for the users to disable it if required. Closes: #564
99 lines
2.1 KiB
JavaScript
99 lines
2.1 KiB
JavaScript
// @flow
|
|
|
|
import {
|
|
SET_ALWAYS_ON_TOP_WINDOW_ENABLED,
|
|
SET_AUDIO_MUTED,
|
|
SET_DISABLE_AGC,
|
|
SET_EMAIL,
|
|
SET_NAME,
|
|
SET_SERVER_URL,
|
|
SET_SERVER_TIMEOUT,
|
|
SET_VIDEO_MUTED
|
|
} from './actionTypes';
|
|
|
|
type State = {
|
|
alwaysOnTopWindowEnabled: boolean,
|
|
disableAGC: boolean,
|
|
email: string,
|
|
name: string,
|
|
serverURL: ?string,
|
|
serverTimeout: ?number,
|
|
startWithAudioMuted: boolean,
|
|
startWithVideoMuted: boolean
|
|
};
|
|
|
|
const username = window.jitsiNodeAPI.osUserInfo().username;
|
|
|
|
const DEFAULT_STATE = {
|
|
alwaysOnTopWindowEnabled: true,
|
|
disableAGC: false,
|
|
email: '',
|
|
name: username,
|
|
serverURL: undefined,
|
|
serverTimeout: undefined,
|
|
startWithAudioMuted: false,
|
|
startWithVideoMuted: false
|
|
};
|
|
|
|
/**
|
|
* Reduces redux actions for features/settings.
|
|
*
|
|
* @param {State} state - Current reduced redux state.
|
|
* @param {Object} action - Action which was dispatched.
|
|
* @returns {State} - Updated reduced redux state.
|
|
*/
|
|
export default (state: State = DEFAULT_STATE, action: Object) => {
|
|
switch (action.type) {
|
|
case SET_ALWAYS_ON_TOP_WINDOW_ENABLED:
|
|
return {
|
|
...state,
|
|
alwaysOnTopWindowEnabled: action.alwaysOnTopWindowEnabled
|
|
};
|
|
|
|
case SET_AUDIO_MUTED:
|
|
return {
|
|
...state,
|
|
startWithAudioMuted: action.startWithAudioMuted
|
|
};
|
|
|
|
case SET_DISABLE_AGC:
|
|
return {
|
|
...state,
|
|
disableAGC: action.disableAGC
|
|
};
|
|
|
|
case SET_EMAIL:
|
|
return {
|
|
...state,
|
|
email: action.email
|
|
};
|
|
|
|
case SET_NAME:
|
|
return {
|
|
...state,
|
|
name: action.name
|
|
};
|
|
|
|
case SET_SERVER_URL:
|
|
return {
|
|
...state,
|
|
serverURL: action.serverURL
|
|
};
|
|
|
|
case SET_SERVER_TIMEOUT:
|
|
return {
|
|
...state,
|
|
serverTimeout: action.serverTimeout
|
|
};
|
|
|
|
case SET_VIDEO_MUTED:
|
|
return {
|
|
...state,
|
|
startWithVideoMuted: action.startWithVideoMuted
|
|
};
|
|
|
|
default:
|
|
return state;
|
|
}
|
|
};
|