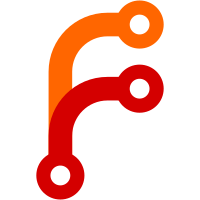
Connects to backend and gets all registered users by role. Enabled editors to see all registered users which wasn't possible.
61 lines
1.7 KiB
TypeScript
61 lines
1.7 KiB
TypeScript
import React from "react";
|
|
import { Paper, Typography } from "@material-ui/core";
|
|
import { makeStyles } from "@material-ui/core/styles";
|
|
import PersonCard from "./PersonCard";
|
|
import { useQuery } from "@apollo/client";
|
|
import {
|
|
BasicPersonResponse,
|
|
GET_PERSONS_SORTED_BY_ROLE,
|
|
GetPersonsSortedByRoleResponse,
|
|
} from "../backend/queries/person";
|
|
|
|
const useStyles = makeStyles((theme) => ({
|
|
root: {
|
|
width: "100%",
|
|
padding: theme.spacing(1),
|
|
marginBottom: theme.spacing(3),
|
|
},
|
|
}));
|
|
|
|
export function UserManagement(): React.ReactElement {
|
|
const classes = useStyles();
|
|
const persons = useQuery<GetPersonsSortedByRoleResponse>(
|
|
GET_PERSONS_SORTED_BY_ROLE
|
|
).data;
|
|
|
|
const convertPersonNodeToPersonCard = (
|
|
person: BasicPersonResponse
|
|
): JSX.Element => {
|
|
return (
|
|
<PersonCard
|
|
key={person.id}
|
|
firstName={person.firstName || ""}
|
|
lastName={person.lastName || ""}
|
|
/>
|
|
);
|
|
};
|
|
|
|
return (
|
|
<div>
|
|
<Paper className={classes.root}>
|
|
<Typography component={"h2"} variant="h6" color="primary" gutterBottom>
|
|
Redaktion
|
|
</Typography>
|
|
{persons?.editors.nodes.map(convertPersonNodeToPersonCard)}
|
|
</Paper>
|
|
<Paper className={classes.root}>
|
|
<Typography component={"h2"} variant="h6" color="primary" gutterBottom>
|
|
Kandidat:innen
|
|
</Typography>
|
|
{persons?.candidates.nodes.map(convertPersonNodeToPersonCard)}
|
|
</Paper>
|
|
<Paper className={classes.root}>
|
|
<Typography component={"h2"} variant="h6" color="primary" gutterBottom>
|
|
Andere registrierte Personen
|
|
</Typography>
|
|
{persons?.users.nodes.map(convertPersonNodeToPersonCard)}
|
|
</Paper>
|
|
</div>
|
|
);
|
|
}
|