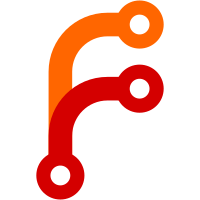
Connects to backend and gets all registered users by role. Enabled editors to see all registered users which wasn't possible.
68 lines
2.9 KiB
TypeScript
68 lines
2.9 KiB
TypeScript
import React from "react";
|
|
import { findByText, render, screen } from "@testing-library/react";
|
|
import { MockedProvider, MockedResponse } from "@apollo/client/testing";
|
|
import { MemoryRouter } from "react-router-dom";
|
|
import { SnackbarProvider } from "notistack";
|
|
import { UserManagement } from "../components/UserManagement";
|
|
import {
|
|
getPersonsSortedByRoleAllFilledMock,
|
|
getPersonsSortedByRoleNoCandidatesMock,
|
|
} from "../backend/queries/person.mock";
|
|
|
|
function renderUserManagementPage(mocks: Array<MockedResponse>) {
|
|
return render(
|
|
<MockedProvider mocks={mocks}>
|
|
<MemoryRouter>
|
|
<SnackbarProvider>
|
|
<UserManagement />
|
|
</SnackbarProvider>
|
|
</MemoryRouter>
|
|
</MockedProvider>
|
|
);
|
|
}
|
|
|
|
describe("The UserManagement page", () => {
|
|
test(" displays all registered persons sorted by role.", async () => {
|
|
renderUserManagementPage(getPersonsSortedByRoleAllFilledMock);
|
|
|
|
const editorsSectionTitle = await screen.findByText(/Redaktion/);
|
|
const editorsSection = editorsSectionTitle.parentElement as HTMLElement;
|
|
const editor = await findByText(editorsSection, /Erika Mustermann/);
|
|
expect(editor).not.toBeNull();
|
|
|
|
const candidatesSectionTitle = await screen.findByText(/Kandidat/);
|
|
const candidatesSection = candidatesSectionTitle.parentElement as HTMLElement;
|
|
const candidate1 = await findByText(candidatesSection, /Max Mustermann/);
|
|
const candidate2 = await findByText(candidatesSection, /Tricia McMillan/);
|
|
expect(candidate1).not.toBeNull();
|
|
expect(candidate2).not.toBeNull();
|
|
|
|
const otherUsersSectionTitle = await screen.findByText(/Andere/);
|
|
const otherUsersSection = otherUsersSectionTitle.parentElement as HTMLElement;
|
|
const otherUser = await findByText(otherUsersSection, /Happy User/);
|
|
expect(otherUser).not.toBeNull();
|
|
});
|
|
|
|
test(" displays all registered persons sorted by role even though there are no candidates.", async () => {
|
|
renderUserManagementPage(getPersonsSortedByRoleNoCandidatesMock);
|
|
|
|
const editorsSectionTitle = await screen.findByText(/Redaktion/);
|
|
const editorsSection = editorsSectionTitle.parentElement as HTMLElement;
|
|
const editor = await findByText(editorsSection, /Erika Mustermann/);
|
|
expect(editor).not.toBeNull();
|
|
|
|
const candidatesSectionTitle = await screen.findByText(/Kandidat/);
|
|
const candidatesSection = candidatesSectionTitle.parentElement as HTMLElement;
|
|
expect(candidatesSection.childElementCount).toBe(1);
|
|
|
|
const otherUsersSectionTitle = await screen.findByText(/Andere/);
|
|
const otherUsersSection = otherUsersSectionTitle.parentElement as HTMLElement;
|
|
const otherUser1 = await findByText(otherUsersSection, /Max Mustermann/);
|
|
const otherUser2 = await findByText(otherUsersSection, /Tricia McMillan/);
|
|
const otherUser3 = await findByText(otherUsersSection, /Happy User/);
|
|
expect(otherUser1).not.toBeNull();
|
|
expect(otherUser2).not.toBeNull();
|
|
expect(otherUser3).not.toBeNull();
|
|
});
|
|
});
|