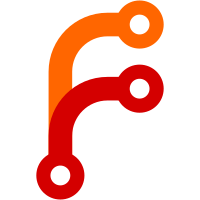
Cache update seems too complicated since there is more than one field "allPerson" when only the cached query witch sorts by role needs to be updated. Automatically refetching the query solves the problem sufficiently good.
103 lines
2.8 KiB
TypeScript
103 lines
2.8 KiB
TypeScript
import React from "react";
|
|
import { IconButton, MenuItem } from "@material-ui/core";
|
|
import Menu from "@material-ui/core/Menu";
|
|
import EditIcon from "@material-ui/icons/Edit";
|
|
import { UPPERCASE_USER_ROLES, UppercaseUserRole } from "../jwt/jwt";
|
|
import { useMutation } from "@apollo/client";
|
|
import {
|
|
CHANGE_ROLE,
|
|
ChangeRoleResponse,
|
|
ChangeRoleVariables,
|
|
} from "../backend/mutations/userRole";
|
|
import { useSnackbar } from "notistack";
|
|
import { GET_PERSONS_SORTED_BY_ROLE } from "../backend/queries/person";
|
|
|
|
interface ChangeRoleProps {
|
|
currentRole: UppercaseUserRole;
|
|
currentUserRowId: number;
|
|
}
|
|
export default function ChangeRole(props: ChangeRoleProps): React.ReactElement {
|
|
const [anchorEl, setAnchorEl] = React.useState<null | HTMLElement>(null);
|
|
const open = Boolean(anchorEl);
|
|
const { enqueueSnackbar } = useSnackbar();
|
|
const otherRoles = UPPERCASE_USER_ROLES.filter(
|
|
(role) => role != props.currentRole
|
|
);
|
|
const handleMenu = (event: React.MouseEvent<HTMLElement>) => {
|
|
setAnchorEl(event.currentTarget);
|
|
};
|
|
const handleClose = () => {
|
|
setAnchorEl(null);
|
|
};
|
|
const [changeRole] = useMutation<ChangeRoleResponse, ChangeRoleVariables>(
|
|
CHANGE_ROLE,
|
|
{
|
|
onCompleted() {
|
|
handleClose();
|
|
},
|
|
onError(e) {
|
|
console.error(e);
|
|
handleClose();
|
|
enqueueSnackbar("Ein Fehler ist aufgetreten, versuche es erneut.", {
|
|
variant: "error",
|
|
});
|
|
},
|
|
refetchQueries: [{ query: GET_PERSONS_SORTED_BY_ROLE }],
|
|
}
|
|
);
|
|
const displayRole = (role: UppercaseUserRole) => {
|
|
switch (role) {
|
|
case "CANDYMAT_CANDIDATE":
|
|
return "zu Kandidat:in machen";
|
|
case "CANDYMAT_EDITOR":
|
|
return "zu RedakteurIn machen";
|
|
case "CANDYMAT_PERSON":
|
|
return "zu Standard User machen";
|
|
}
|
|
};
|
|
return (
|
|
<React.Fragment>
|
|
<IconButton
|
|
aria-label="role of current user"
|
|
aria-controls="change-role"
|
|
aria-haspopup="true"
|
|
onClick={handleMenu}
|
|
color="inherit"
|
|
>
|
|
<EditIcon />
|
|
</IconButton>
|
|
<Menu
|
|
id="change-role"
|
|
anchorEl={anchorEl}
|
|
anchorOrigin={{
|
|
vertical: "top",
|
|
horizontal: "right",
|
|
}}
|
|
keepMounted
|
|
transformOrigin={{
|
|
vertical: "top",
|
|
horizontal: "right",
|
|
}}
|
|
open={open}
|
|
onClose={handleClose}
|
|
>
|
|
{otherRoles.map((role) => (
|
|
<MenuItem
|
|
key={role}
|
|
onClick={() => {
|
|
changeRole({
|
|
variables: {
|
|
personRowId: props.currentUserRowId,
|
|
newRole: role,
|
|
},
|
|
});
|
|
}}
|
|
>
|
|
{displayRole(role)}
|
|
</MenuItem>
|
|
))}
|
|
</Menu>
|
|
</React.Fragment>
|
|
);
|
|
}
|