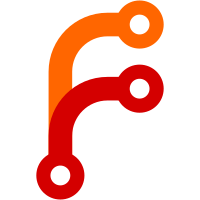
Return the whole person object on calling the changeRole mutation. This enables the apollo cache in the frontend to automatically update the role of the given user. Furthermore, made all create function statements idempotent by deleting them first if existing.
58 lines
1.2 KiB
TypeScript
58 lines
1.2 KiB
TypeScript
import { gql } from "@apollo/client";
|
|
import { UppercaseUserRole } from "../../jwt/jwt";
|
|
|
|
export const BasicPersonFragment = gql`
|
|
fragment BasicPersonFragment on Person {
|
|
id
|
|
rowId
|
|
firstName
|
|
lastName
|
|
role
|
|
}
|
|
`;
|
|
|
|
export interface BasicPersonResponse {
|
|
id: string;
|
|
rowId: number;
|
|
firstName: string | null;
|
|
lastName: string | null;
|
|
role: UppercaseUserRole;
|
|
__typename: "Person";
|
|
}
|
|
|
|
export const GET_PERSONS_SORTED_BY_ROLE = gql`
|
|
query AllPeople {
|
|
editors: allPeople(condition: { role: CANDYMAT_EDITOR }) {
|
|
nodes {
|
|
...BasicPersonFragment
|
|
}
|
|
}
|
|
candidates: allPeople(condition: { role: CANDYMAT_CANDIDATE }) {
|
|
nodes {
|
|
...BasicPersonFragment
|
|
}
|
|
}
|
|
users: allPeople(condition: { role: CANDYMAT_PERSON }) {
|
|
nodes {
|
|
...BasicPersonFragment
|
|
}
|
|
}
|
|
}
|
|
${BasicPersonFragment}
|
|
`;
|
|
|
|
export interface GetPersonsSortedByRoleResponse {
|
|
editors: {
|
|
nodes: Array<BasicPersonResponse>;
|
|
__typename: "PeopleConnection";
|
|
};
|
|
candidates: {
|
|
nodes: Array<BasicPersonResponse>;
|
|
__typename: "PeopleConnection";
|
|
};
|
|
users: {
|
|
nodes: Array<BasicPersonResponse>;
|
|
__typename: "PeopleConnection";
|
|
};
|
|
}
|